Python 圖像風格遷移實現
在當今的數碼時代,人工智慧和深度學習技術的快速發展,使得我們能夠實現許多過去難以想像的創新應用。其中,圖像風格遷移(Neural Style Transfer)就是一個典型的例子。圖像風格遷移技術可以將一幅圖片的內容和另一幅圖片的藝術風格結合起來,生成一幅新的圖像。這項技術不僅在藝術創作領域引起了廣泛關注,也在商業設計、廣告等領域展現出了巨大的潛力。
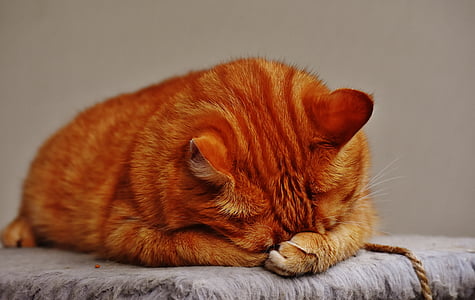
圖像風格遷移的核心原理基於卷積神經網絡(Convolutional Neural Network, CNN)。CNN 是一種專門處理圖像數據的神經網絡結構,具有強大的特徵提取和模式識別能力。在圖像風格遷移中,CNN 用於分別提取內容圖片和風格圖片的特徵,然後通過優化方法將這些特徵融合到一起,最終生成既保留原始圖片內容,又具有目標風格的全新圖像。
要實現圖像風格遷移,我們需要以下幾個基本步驟:
準備內容圖片和風格圖片。
建立並訓練卷積神經網絡。
定義損失函數,計算內容損失和風格損失。
使用優化算法,最小化損失函數,生成最終圖像。
下面,我們將使用 Python 語言和流行的深度學習框架 TensorFlow,來實現一個簡單的圖像風格遷移模型。
我們需要安裝必要的庫和模組:
!pip install tensorflow
!pip install pillow
接下來,我們導入所需的模組:
import tensorflow as tf
import numpy as np
import PIL.Image
import matplotlib.pyplot as plt
接著,我們定義一個函數,用於加載和處理圖片:
def load_img(path_to_img):
max_dim = 512
img = PIL.Image.open(path_to_img)
long = max(img.size)
scale = max_dim / long
img = img.resize((round(img.size[0] * scale), round(img.size[1] * scale)), PIL.Image.ANTIALIAS)
img = np.array(img)
# We need to broadcast the image array such that it has a batch dimension
img = np.expand_dims(img, axis=0)
return img
def imshow(image, title=None):
if len(image.shape) > 3:
image = tf.squeeze(image, axis=0)
plt.imshow(image)
plt.title(title)
現在,我們加載內容圖片和風格圖片:
content_path = 'path_to_your_content_image.jpg'
style_path = 'path_to_your_style_image.jpg'
content_image = load_img(content_path)
style_image = load_img(style_path)
plt.subplot(1, 2, 1)
imshow(content_image, 'Content Image')
plt.subplot(1, 2, 2)
imshow(style_image, 'Style Image')
plt.show()
在這個示例中,我們使用了自己的圖片路徑來加載內容圖片和風格圖片。讀者可以替換 path_to_your_content_image.jpg 和 path_to_your_style_image.jpg 為實際圖片的路徑。
接下來,我們使用預訓練的 VGG19 模型來提取圖片的特徵。VGG19 是一種深層卷積神經網絡,在圖像分類和特徵提取方面表現優異。TensorFlow 提供了方便的接口來加載 VGG19 模型:
def load_vgg_model():
vgg = tf.keras.applications.VGG19(include_top=False, weights='imagenet')
vgg.trainable = False
return vgg
def get_feature_representations(model, content_image, style_image):
content_layers = ['block5_conv2']
style_layers = ['block1_conv1', 'block2_conv1', 'block3_conv1', 'block4_conv1', 'block5_conv1']
num_content_layers = len(content_layers)
num_style_layers = len(style_layers)
content_outputs = [model.get_layer(name).output for name in content_layers]
style_outputs = [model.get_layer(name).output for name in style_layers]
model_outputs = content_outputs + style_outputs
extract_model = tf.keras.Model(inputs=model.inputs, outputs=model_outputs)
content_features = extract_model(content_image)
style_features = extract_model(style_image)
content_features = [content_features[i] for i in range(num_content_layers)]
style_features = [style_features[i + num_content_layers] for i in range(num_style_layers)]
return content_features, style_features
vgg = load_vgg_model()
content_features, style_features = get_feature_representations(vgg, content_image, style_image)
在這裡,我們定義了一個函數 load_vgg_model() 來加載 VGG19 模型,並使用 get_feature_representations() 函數來提取內容圖片和風格圖片的特徵。
接下來,我們需要定義損失函數來計算內容損失和風格損失。內容損失度量生成圖像和內容圖像之間的相似度,而風格損失則度量生成圖像和風格圖像之間的相似度。我們使用 Gram 矩陣來計算風格損失,Gram 矩陣是一種常用的統計方法,用於描述風格圖像中不同特徵之間的關係。
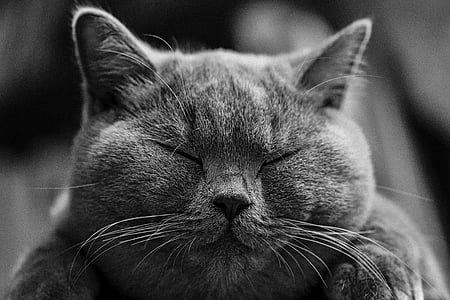
def compute_content_loss(base_content, target):
return tf.reduce_mean(tf.square(base_content - target))
def gram_matrix(input_tensor):
channels = int(input_tensor.shape[-1])
a = tf.reshape(input_tensor, [-1, channels])
n = tf.shape(a)[0]
gram = tf.matmul(a, a, transpose_a=True)
return gram / tf.cast(n, tf.float32)
def compute_style_loss(base_style, gram_target):
height, width, channels = base_style.get_shape().as_list()
gram_style = gram_matrix(base_style)
return tf.reduce_mean(tf.square(gram_style - gram_target))
接著,我們定義總損失函數,這個損失函數是內容損失和風格損失的加權和:
```python
def computeloss(model, lossweights, initimage, gramstylefeatures, contentfeatures):
modeloutputs = model(initimage)
contentoutputfeatures = modeloutputs[:len(contentfeatures)]
styleoutputfeatures = modeloutputs[len(contentfeatures):]
content_score = 0
style_score = 0
weight_per_content_layer = 1.0 / float(len(content_features))
for target_content, comb_content in zip(content_features, content_output_features):
content_score += weight_per_content_layer * compute_content_loss(comb_content, target_content)
weight_per_style_layer = 1.0 / float(len(gram_style_features))
for target_style, comb_style in zip(gram_style_features, style_output_features):
style_score += weight_per_style_layer * compute_style_loss(comb
試想,一個沒有計算機的世界將會是何等模樣?從簡單的文字編輯到複雜的科學計算,從社交媒體的即時通訊到全球經濟的精準調控,計算機無處不在。本文將帶您走進計算機的世界,了解其運行機制與應用價值。
发表评论